SDK Sandbox
SDK preprod
ComexposiumConnect SSO
- SSO
- Script loader
- HTML Code
- Example
- Methods
- Open the login interface
- Open the account creation interface
- Open the forgotten password interface
- Open the generic messages interface
- Authenticate a user
- Create a new user
- Check if the user is logged in
- Check the user status
- Disconnect the user
- Events
- comexposiumConnectLoaded
- comexposiumConnectLogged
- comexposiumConnectLogout
- comexposiumConnectTokenExpired
- comexposiumConnectRegisterOk
- Retrieve user information
- Errors Codes
ComexposiumConnect SSO
The ComexposiumConnect SSO widget is used to simplify the experience of our users as they move from one service to another.
You can check our API. There is a sandbox plugged on the pre-preproduction environment.
This documentation provides examples on how to install, use and get some basic informations about comexposiumConnect.
If you are a third party user, you will probably receive an account with specific rights allowing you to have specific access to the API. This account will be flagged as “thirdPartyAccount” and will have specific rights only for API purpose.
Using a "thirdPartyAccount" account won't work on frontend. Please consider creating a brand new one in order to use it as a regular user on frontend.
SSO
The principle
Single Sign-On (SSO) is the feature that allows a user to authenticate once for the duration of a session regardless of the number of applications that require authentication.
Then, users can directly access their data, without enter a new pair of username / password again.
The goal
To work, the ComexposiumConnect widget must be installed on all pages of your application / platform. Once the widget is installed, you can create an account and test SSO connexion.
How it works
Once the widget is correctly installed and initialized, the transition from a Comexposium platform to your application / platform must be possible without the user need to connect again.
To ensure the operation from one of the Comexposium platforms to your application / platform, you have at your disposal all the methods offered by the Comexposium API in addition to those available below.
Changing domain
When you are connected on a domain name A and you want to switch to domain name B, you will see the switch popin that explains you that the SSO connexion works as well:
You can accept by connecting threw the SSO connexion, or you can refuse and disconnect.
Script loader
After jQuery loaded on your page, you will need to load the following script that comes in 2 environments depending on the progress of your project:
Pre-production:
<script src="https://connect.preprod.comexposium-webservices.com/js/loader.min.js"></script>
Production:
<script src="https://connect.prod.comexposium-webservices.com/js/loader.min.js"></script>
In order to use the ComexposiumConnect widget, You first need to load jQuery.
We encourage third party applications to use at least jQuery 2.0, but we strongly recommend to use a more recent version (3.x).
As a security, if we don't detect jQuery or if the version is older than 2.0, we dynamically load jQuery 3.1.1 without taking hand on the dollar ($) Javascript var. It allows you to still use your version or your library.
The new version is available in the var:
window.cxpm.jQuery
Once the loader.min.js script is loaded and authorized by the Comexposium Team, you can access to the global variables comexposiumCap and comexposiumConnect.
You can also check which version of the loader and SDK you work with:
cxpm.version.useraccount |
Now, you just have to include the HTML Code in your page to load the widget.
HTML Code
The values you have to put in the HTML attributes are specific to each event, each event and each session. They will be communicated to you directly by the Comexposium Team.
The Comexposium Team will also have to allow all the domain names you call, otherwise you will have the following errors in the javascript console:
wrong application token
CORS: Response to preflight request doesn't pass access control check
Once all your domain names have been authorized by Comexposium, and the loader.min.js script as well as your version of jQuery are correctly loaded in your application / platform, you can add the following code in the body part of your HTML code:
<div id="cxpmClientAccountWidget" |
This is a simple div with 4 attributes. Each of these attributes refers to the event:
- [SHOWROOM_NAME] : The name of the show related to your use.
- [SHOWROOM_SESSION] : The name of the session related to your use
- [YOUR_APPLICATION_ID] : Your application id in the ComexposiumConnect environment.
This one is unique whatever the showroom or the session.
It also differs depending on whether you are in production or pre-production. - [LANGUAGE] :It can take 2 possible values : "fre-FR" or "eng-GB"
These elements will be sent to you by the Comexposium Team.
Full example
Here is an example using the pre-production environment, and having been authorized for the domain name: http://127.0.0.1:63569. You can test it by launching a local web server (especially thanks to this free chrome app) on the http port 63569 :
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Exemple</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js" charset="utf-8"></script> </head> <body> <div id="cxpmClientAccountWidget" data-salon="exemple" data-sessionSalon="exemple_2018" data-application="2a6d06fc8089bbff0889aadc02d4a14ac1bdd03a" data-language="eng-GB" > <!-- Nothing here --> </div> |
Methods
Most of the methods below are also available on the ComexposiumConnect API. You'll also find API routes for the authentication, the user management, the third-party application management, and the selections management.
Open the login interface
Here is an example below that opens the SSO login interface:
comexposiumCap.openCap('login');
|
Open the account creation interface
Here is an example below that calls the SSO account creation interface:
comexposiumCap.openCap('register');
|
It is also possible to switch from the login interface to the account creation interface directly from the button "Create an account" in the footer of the widget:
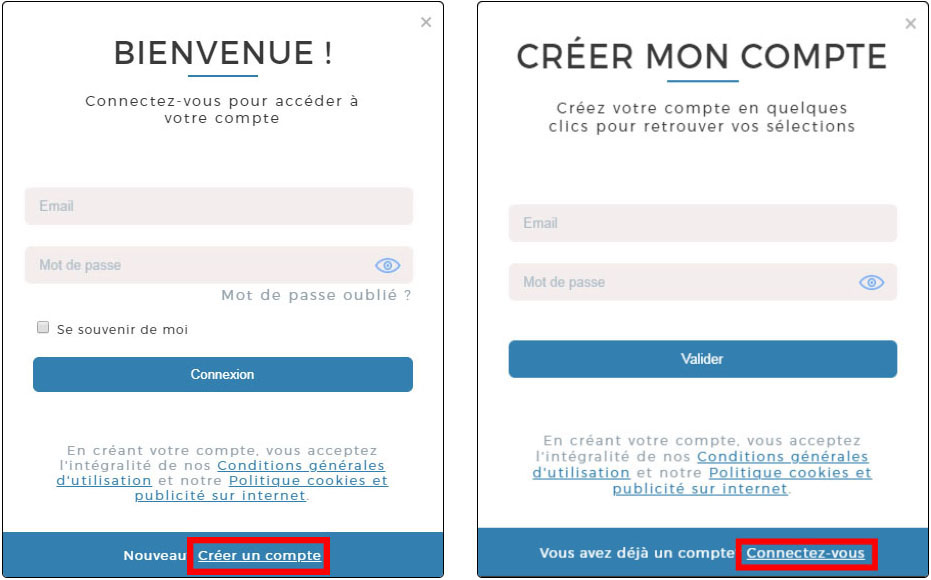
On the pre-production environment, all the emails sent (account creation, password forgotten, newsletters... ) are redirected to our internal mailbox [email protected]
You can request access to your contact at Comexposium.
Open the forgotten password interface
Here is an example below that calls the password reset interface:
comexposiumCap.openCap('request-password');
|
Resetting the password fires an email containing the password reset link like this:
https://myurl.com/#reset-password?token=XXXXXX
You will need to make sure that the main URL that you declare to Comexposium will not contain any redirection and make sure that the ComexposiumConnect SSO widget is installed too.
Remember that emails sent in the pre-production environment are intercepted and automatically landed into this email address:
[email protected].
Open the generic messages interface
Here is an example below that calls the generic interface you can use to display messages:
comexposiumCap.openGenericCap('Title', 'Description');
|
Authenticate a user
To authenticate a user, you can use the following method:
comexposiumConnect.loginUser("email", "password", false, function(){ // Here I can check my statusCode and do whatever I want if (result.statusCode === 0){ // login successful } else { // login failed console.log(result.statusCode, result.message); } }); |
The third parameter is a boolean which corresponds to the stayConnected variable. The last parameter is a callback and is not mandatory.
Create a new user
To create a new user, you can use the following method:
comexposiumConnect.createUser("email", function(){ // Here I can check my statusCode and do whatever I want if (result.statusCode === 0){ // create successful } else { // create failed console.log(result.statusCode, result.message); } }); |
Using this method is equivalent to using this endpoint of our API: /_plugin/Comexposium/user/create.
An activation account email will be automatically sent to the email address added as a first parameter. This email contains a link that will allow this user to create his password.
On the pre-production environment, all the emails sent (account creation, password forgotten, newsletters... ) are redirected to our internal mailbox [email protected]
You can request access to your contact at Comexposium.
Check if the user is logged in
You can check if a user is logged in. This method returns a boolean.
true: the user is logged in
false: the user is not logged in
comexposiumCap.isUserLogged();
|
Check the user status
You can get the user status. This method returns an array with all user status.
If the user have no status, or if he isn't connected, the result will be an empty array.
You can add or remove any status by using our API
comexposiumConnect.getUserTypes(function(listOfUserType){
console.log(listOfUserType)
});
|
Disconnect a user
You can disconnect the user by doing:
comexposiumCap.disconnectUser(list);
|
Javascript Events
When the library is properly loaded, it fires certain events to ease your workflow. Keep in mind that the widget can creating a JavaScript variable named window.cxpm.jQuery with the required version of jQuery. Outside the comexposiumConnectLoaded event, all events are triggered with window.cxpm.jQuery.
comexposiumConnectLoaded
When the library is fully loaded, an event is triggered to inform you that everything is ready. You can listen to your custom events from the moment when the widget is ready, this event will be triggered from the global scope jQuery ($ or jQuery):
$(document).on("comexposiumConnectLoaded", function(){
Execute something
window.cxpm.jQuery(document).on("MON_EVENEMENT",function(){
Listen to a personalized event
});
});
|
comexposiumConnectLogged
This event is triggered when the user is connected in SSO to ComexposiumConnect. This can happen when the page is loaded (the user is already connected to the system) or when submitting the login form:
$(document).on("comexposiumConnectLogged", function(){
Execute something
});
|
comexposiumConnectLogout
This event is triggered when the user is disconnected. This can happen when the user has been disconnected in an other tab, or in an other application:
$(document).on("comexposiumConnectLogout", function(){
Execute something
});
|
comexposiumConnectLogged
This event is triggered when the token expired. Remember that the JWT token has a validated date
$(document).on("comexposiumConnectTokenExpired", function(){
Execute something
});
|
comexposiumConnectRegisterOk
This event indicates that the user closed the interface after creating an account. The callback can take 2 parameters, the event and the e-mail address used to create the account:
window.cxpm.jQuery(document).on("comexposiumConnectRegisterOk", function(event, email){
An account has been created and we can get the email
});
|
Retrieve user information
The user can be recognized and logged in automatically via the SSO cookie or he can log in using the ComexposiumConnect login widget. Then, it is possible to get the user's email, the JWT token and we can know if the connected email is an exhibitor, a vip or a speaker on the current session.
To perform this, you can listen to the jQuery event named "comexposiumConnectLogged" which can take 4 parameters including email:
$(document).on("comexposiumConnectLogged", function(event, email, token, isExhibitor){
console.log(email, token, isExhibitor);
});
|
Try it by sign in with the button below and open your browser console:
To check the information on the server side, you can also use our API to make sure that the user's token or email is valid.
Error codes
Name |
Code |
Description |
updateUserError |
1 |
Error updating user informations |
updateUserSuccess |
0 |
Success |
updateUserWrongCredentials |
2 |
Wrong credentials, refers to the documentation |
updateUserSearchToken |
3 |
2 or more tokens found |
updateUserNotFound |
4 |
Token not found |
getDefaultLangugeError |
161 |
Error fetching the default language |
requestRegeneratePasswordWrongCredentials |
5 |
Wrong credentials, refers to the documentation |
requestRegeneratePasswordError |
6 |
Error searching email |
requestRegeneratePasswordSuccess |
0 |
Success |
requestRegeneratePasswordNotFound |
7 |
Email not found |
regeneratePasswordWrongCredentials |
8 |
Wrong credentials, refers to the documentation |
regeneratePasswordError |
9 |
Error searching token |
regeneratePasswordSuccess |
0 |
Success |
regeneratePasswordNotFound |
10 |
Token not found |
checkFirstLoginTokenError |
11 |
Error searching token |
checkFirstLoginTokenNotFound |
12 |
Token not found |
checkFirstLoginTokenWrongCredentials |
13 |
Wrong credentials, refers to the documentation |
checkFirstLoginTokenSuccess |
0 |
Success |
checkFirstLoginAlreadyValidated |
35 |
Account already validated |
checkFirstLoginOutdated |
36 |
Token outdated |
checkFirstLoginErrorGetUserData |
183 |
- |
checkCookieNotOk |
150 |
There is no SSO Cookie |
checkCookieSuccess |
0 |
Success |
onLoginMailNotFound |
87 |
Mail not found |
onLoginError |
88 |
Internal error searching for email |
createUserMissingCredentials |
131 |
Wrong credentials, refers to the documentation |
createUserSearch |
132 |
Error searching email |
createUserSetupToken |
133 |
Error creating the token for user |
createUserError |
134 |
Error creating the user |
createUserUpdateError |
135 |
- |
createUserMailExists |
143 |
Email already exists |
deleteTemporaryUserErrorGetUser |
157 |
Error fetching the user |
deleteTemporaryUserErrorUpdate |
158 |
- |
deleteTemporaryUserMissingCredentials |
159 |
Wrong credentials, refers to the documentation |
deleteTemporaryUserErrorLanguage |
160 |
- |
deleteTemporaryUserSuccess |
0 |
Success |
deleteTemporaryUserErrorMail |
162 |
Error fetching email in order to send deleted email |
addItemMySelectionError |
15 |
Error creating / updating the selection |
addItemMySelectionSuccess |
0 |
Success |
addItemMySelectionBadToken |
16 |
- |
addItemMySelectionAlreadyIn |
17 |
Item already in the selection |
addItemMySelectionMultipleSelections |
37 |
Internal error with multiple selection for same userId |
addItemMySelectionWrongCredentials |
26 |
Wrong credentials, refers to the documentation |
addItemMySelectionNoLanguage |
144 |
- |
addItemMySelectionErrorLanguage |
145 |
- |
addItemMySelectionErrorInformationsItem |
146 |
- |
addItemMySelectionUpdateError |
147 |
- |
addItemMySelectionCreateError |
148 |
- |
getMySelectionError |
18 |
Impossible to give the selection |
getMySelectionSuccess |
0 |
Success |
getMySelectionNotFound |
19 |
- |
getMySelectionWrongCredentials |
20 |
Wrong credentials, refers to the documentation |
getMySelectionMultipleSelection |
21 |
Multiple selection for the same user |
getMySelectionWrongToken |
22 |
- |
deleteItemMySelectionWrongCredentials |
23 |
Wrong credentials, refers to the documentation |
deleteItemMySelectionError |
24 |
Internal server error |
deleteItemMySelectionBadToken |
25 |
- |
deleteItemMySelectionNotFound |
27 |
There is no selection for the given user |
deleteItemMySelectionMultipleSelection |
28 |
Multiple selection for the same user |
deleteItemMySelectionSuccess |
0 |
Success |
getLastItemsError |
29 |
Error retrieving last items in selection |
getLastItemsNotFound |
30 |
No selection for user |
getLastItemsSuccess |
0 |
Success |
getLastItemsMultipleSelections |
31 |
Multiple selections for user |
getLastItemsWrongToken |
32 |
|
getLastItemsWrongCredentials |
33 |
Wrong credentials, refers to the documentation |
importNewSelectionMissingCredentials |
91 |
Wrong credentials, refers to the documentation |
importNewSelectionErrorSearching |
92 |
Error searching the user |
importNewSelectionUserNotExists |
93 |
Email doesn’t exists |
importNewSelectionCreateError |
94 |
Error creating the selection |
importNewSelectionUpdateError |
95 |
Error updating the selection |
importNewSelectionMultipleSelection |
96 |
Multiple selections for email |
importNewSelectionSuccess |
0 |
Success |
retrieveItemsInformationsMissingCredentials |
151 |
Wrong credentials, refers to the documentation |
importCatalogWrongCredentials |
34 |
Wrong credentials, refers to the documentation |
getSettingsError |
38 |
Error getting profile settings |
getSettingsSuccess |
0 |
Success |
getSettingsMissingCredentials |
41 |
Wrong credentials, refers to the documentation |
getWordingError |
39 |
Error when fetching wordings |
getWordingSuccess |
0 |
Success |
getWordingMissingCredentials |
40 |
Wrong credentials, refers to the documentation |
getCountriesError |
142 |
Error fetching the countries |
getLabelsSuccess |
0 |
Success |
updateUserInformationsError |
42 |
Internal server error |
updateUserInformationsSuccess |
0 |
Success |
updateUserInformationsMissingCredentials |
43 |
Wrong credentials, refers to the documentation |
updateUserInformationsErrorGetUserSalon |
48 |
Error fetching user informations lvl 1 |
updateUserInformationsErrorGetUserSession |
49 |
Error fetching user informations lvl 2 |
updateUserInformationsErrorCreateUserSalon |
50 |
Error creating user informations lvl 1 |
updateUserInformationsErrorCreateUserSession |
51 |
Error creating user informations lvl 2 |
updateUserInformationsErrorUpdateUserSalon |
52 |
Error updating user informations lvl 1 |
updateUserInformationsErrorUpdateUserSession |
53 |
Error updating user informations lvl 2 |
getProfileUserError |
44 |
Error retrieving all lvl informations of the user |
getProfileUserErrorSalon |
45 |
- |
getProfileUserErrorSessionSalon |
46 |
- |
getUserInformationsMissingCredentials |
47 |
Wrong credentials, refers to the documentation |
getProfileUserSuccess |
0 |
Success |
updatePasswordFromProfileMissingCredentials |
88 |
Wrong credentials, refers to the documentation |
updatePasswordFromProfileError |
89 |
Internal server error |
updatePasswordFromProfileNotFound |
90 |
Email not found |
updatePasswordFromProfileSuccess |
0 |
Success |
getFirstNameMissingCredentials |
98 |
Wrong credentials, refers to the documentation |
getFirstNameMailNotExists |
99 |
- |
getFirstNameError |
100 |
Error fetching user informations |
getFirstNameSuccess |
0 |
Success |
getFirstNameNotExists |
110 |
Firstname is not saved in user data |
getUserFromUserIdError |
101 |
Error seaching user by email |
checkRegeneratePasswordTokenWrongCredentials |
163 |
Wrong credentials, refers to the documentation |
checkRegeneratePasswordTokenError |
164 |
Error searching for token |
checkRegeneratePasswordTokenNotFound |
165 |
Token not found |
checkRegeneratePasswordTokenSuccess |
0 |
Success |
getStartingInformationsMissingCredentials |
54 |
Wrong credentials, refers to the documentation |
getStartingInformationsError |
55 |
Error retrieving global user account informations |
getStartingInformationsSuccess |
0 |
Success |
onCreateUserEmailExists |
84 |
- |
onCreateUserError |
85 |
- |
onCreateUserNoMailTemplate |
86 |
- |
createNeededDocumentsGetUserData |
166 |
Internal server error |
createNeededDocumentsCreateUserData |
167 |
Internal server error |
renderLoggedHtmlError |
103 |
Error generating html |
renderLoggedHtmlSuccess |
0 |
Success |
getSalonConfigError |
102 |
Error fetching lvl 1 informations |
getSessionSalonConfigError |
103 |
Error fetching lvl 2 informations |
updateNewsletterMissingCredentials |
104 |
Wrong credentials, refers to the documentation |
updateNewsletterErrorGetUser |
105 |
- |
updateNewsletterErrorGetUserData |
106 |
- |
updateNewsletterErrorCreateData |
107 |
Error saving data for user |
updateNewsletterErrorUpdateData |
108 |
Error updating data for user |
updateNewsletterSuccess |
0 |
Success |
updateNewsletterError |
109 |
Internal server error |
getNewsletterMissingCredentials |
113 |
Wrong credentials, refers to the documentation |
getNewsletterError |
114 |
Internal server error |
getNewsletterErrorGetUserData |
115 |
Error fetching data for user |
getNewsletterSuccess |
0 |
Success |
getConfigNewsletterMissingCredentials |
116 |
Wrong credentials, refers to the documentation |
getConfigNewsletterError |
117 |
Error fetching informations about newsletter |
getConfigNewsletterNoConfig |
118 |
No config saved about newsletter |
getConfigNewsletterSuccess |
0 |
Success |
createNewsletterAnonymousUserError |
119 |
Error creating user informations when saving newsletter |
createNewsletterAnonymousUserNoMailTemplate |
120 |
- |
createNewsletterAnonymousUserSuccess |
0 |
Success |
AddNewsletterMissingCredentials |
121 |
Wrong credentials, refers to the documentation |
AddNewsletterErrorSearch |
122 |
Internal server error |
AddNewsletterErrorCreate |
123 |
Error creating the user when registering newsletter |
getSalonRefError |
124 |
No reference url found to replace {#URL#} |
registerNewsletterSearchToken |
125 |
Error searching newsletter token |
registerNewsletterMultipleToken |
126 |
Multiple newsletter token |
registerNewsletterMissingCredentials |
127 |
Wrong credentials, refers to the documentation |
registerNewsletterUpdateError |
128 |
Error updating newsletter data for user |
registerNewsletterAlreadyValidated |
129 |
Newsletter already validated with this token |
registerNewsletterSuccess |
0 |
Success |
checkNewsletterAlreadyData |
130 |
User already register as anonymous in the base |
SSOGetUserMissingUserEmail |
137 |
No email given to the REST API |
SSOGetUserInternalError |
138 |
Internal server error |
SSOGetUserMailNotExists |
139 |
Email doesn’t exists |
SSOGetUserWrongApplicationConfiguration |
140 |
ThirdParty doesn’t exists |
SSOGetUserSuccess |
0 |
Success |
SSOGetUserInvalidAppToken |
149 |
AppToken access is revocated |
SSOGetUserErrorGet |
171 |
Error fetching the current user |
SSOGetUserThirdPartyError |
172 |
Error fetching and checking appToken |
SSOGetUserSearchUser |
173 |
Error searching user |
getUserForThirdPartyMissingCredentials |
141 |
Wrong credentials, refers to the documentation |
checkThirdPartyTokenErrorSearch |
152 |
Internal server error |
checkThirdPartyTokenNotFound |
153 |
Third party token not found |
checkThirdPartyTokenAuthorized |
154 |
- |
checkThirdPartyTokenMissingCredentials |
155 |
Wrong credentials, refers to the documentation |
checkThirdPartyTokenNotAuthorized |
156 |
AppToken not authorized on the given url |
checkThirdPartyNoOrigin |
196 |
No Origin HTTP header |
checkThirdPartyTokenSuccess |
0 |
Success |
updateUserInfosThirdMissingCredentials |
174 |
Wrong credentials, refers to the documentation |
updateUserInfosThirdUserNotExists |
175 |
Email not found |
updateUserInfosThirdSearchUserError |
176 |
Error searching for email |
updateUserInfosThirdUpdateError |
177 |
Error saving given data |
updateUserInfosThirdGetUserDataError |
178 |
Error fetching user data |
updateUserInfosThirdSuccess |
0 |
Success |
changeUserEmailMissingCredentials |
188 |
Wrong credentials, refers to the documentation |
changeUserEmailOldEmailNotExists |
189 |
Old email doesn’t exists |
changeUserEmailNewEmailAlreadyExists |
190 |
New email already exists |
changeUserEmailNotNormalUser |
191 |
Trying to change email of a not normal user |
changeUserEmailSuccess |
0 |
Success |
checkUserExhibitorMissingCredentials |
198 |
Wrong credentials, refers to the documentation |
checkUserExhibitorNotFound |
199 |
Email not found |
checkUserExhibitorError |
200 |
Internal server error |
checkUserCredentialsMissingCredentials |
201 |
Wrong credentials, refers to the documentation |
checkUserCredentialsInternalError |
202 |
Internal server error |
exportMissingCredentials |
168 |
Wrong credentials, refers to the documentation |
exportWrongTimestamp |
169 |
Given timestamp is less or equal 0 |
relaunchInactiveMissingCredentials |
192 |
Wrong credentials, refers to the documentation |
relaunchInactiveError |
193 |
Internal server error |
checkAuthorizedLanguagesError |
170 |
Error checking given language is allowed for a showroom |
getLoggedUserError |
179 |
- |
getApplicationUserError |
180 |
Error fetching third party informations |
applicationNotAuthorized |
181 |
Third party is not authorized |
newsletterNoMainNewsletter |
182 |
No main newsletter configured |
getUserDataError |
184 |
Error fetching userData |
updateUserDataError |
185 |
Error updating userData |
createUserDataError |
186 |
Error creating userData |
getMailTemplateNotFound |
187 |
No email template found |
missingToken |
194 |
- |
checkFirstLoginNoMainNewsletter |
195 |
error fetching main newsletter |
wrongItemTypeForSelection |
197 |
Item type for selection is not allowed |
deletedUser |
202 |
Trying to log a temporary deleted user |
getUserError |
203 |
Error fetching user |